REST API Automation Testing using Java
In this post we will see how to perform automation testing of REST API using Java. We will use the below sample API of get employees list.
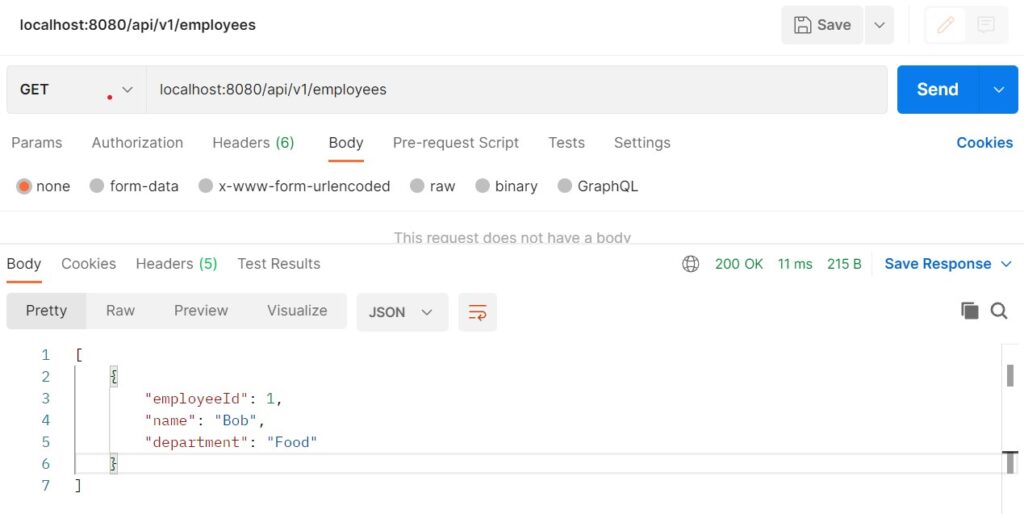
To perform the automation we will use the Java’s HttpURLConnection. HTTPURLConnection from java.net package is a URLConnection along with support for HTTP-specific features. It can be used to send HTTP requests. Using HTTPURLConnection have implemented a method to send GET request and get the response. Similar implementation can be done to automate other HTTP methods.
public class Solution {
private static HttpURLConnection connection = null;
public static String sendGetRequest(String url) {
URL getURL;
StringBuffer response = new StringBuffer();
try {
getURL = new URL(url);
connection = (HttpURLConnection) getURL.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
connection.disconnect();
}
return response.toString();
}
}
Then we will write a test to verify the expected and actual response are equal.
class SolutionTest {
@Test
void test() {
assertEquals("[{\"employeeId\":1,\"name\":\"Bob\",\"department\":\"Food\"}]", Solution.sendGetRequest("http://localhost:8080/api/v1/employees"));
}
}
Leave a Reply