Serverless Functions with Spring Cloud Function, AWS Lambda
Spring Cloud Function is a project within the Spring ecosystem that allows developers to build serverless applications using Spring Boot and deploy them to various cloud platforms, including AWS Lambda.
AWS Lambda is a serverless compute service provided by Amazon Web Services. It allows to run code without provisioning or managing servers. With Spring Cloud Function, can write functions using Spring’s programming model and deploy them to AWS Lambda.
Spring Cloud Function uses core functional interfaces provided by Java. We can create functions as Supplier (produces a result without any input), Consumer (consumes an input and produces no result), and Function (transforms an input to an output).
Lets go through the steps required to create the Serverless function. To get started create a Spring boot project with the below dependencies
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-function-context</artifactId> </dependency> <dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-lambda-java-events</artifactId> <version>${aws-lambda-events.version}</version> </dependency> <dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-lambda-java-core</artifactId> <version>1.1.0</version> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-function-adapter-aws</artifactId> </dependency>
Define the function as beans using @Bean
annotations
@SpringBootApplication public class CloudFunctionsLambdaApplication { public static void main(String[] args) { SpringApplication.run(CloudFunctionsLambdaApplication.class, args); } @Bean public Function<String, String> uppercase() { return value -> value.toUpperCase(); } }
The next important step is to build the jar which needs to be uploaded to AWS lambda. We will use the maven shade plugin to build a fat jar including all the dependencies.
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-deploy-plugin</artifactId> <configuration> <skip>true</skip> </configuration> </plugin> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <dependencies> <dependency> <groupId>org.springframework.boot.experimental</groupId> <artifactId>spring-boot-thin-layout</artifactId> <version>1.0.28.RELEASE</version> </dependency> </dependencies> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <configuration> <createDependencyReducedPom>false</createDependencyReducedPom> <shadedArtifactAttached>true</shadedArtifactAttached> <shadedClassifierName>aws</shadedClassifierName> </configuration> </plugin> </plugins> </build>
Next lets create the lambda function from AWS console.
Create a Lambda Function:
- Go to the AWS Lambda console.
- Click on “Create function”.
- Choose the authoring method (either from scratch, using a blueprint, or by deploying an AWS Serverless Application Repository application).
- Enter the function name, runtime(Java in this case), and other configuration details.
- For the code upload the jar
- For the handler specify org.springframework.cloud.function.adapter.aws.FunctionInvoker::handleRequest which is a generic request handler.
Test the Function:
- Use the AWS Lambda console to test your function by providing sample input or trigger it using test events.
Invoke the Function:
- Trigger the function manually through the AWS Management Console, use AWS SDKs, AWS CLI, or configure triggers such as API Gateway, S3 events, etc., to invoke Lambda function automatically based on certain events.
- When function URL is configured, it can be used to invoke the function through a browser, curl, Postman, or any HTTP client.
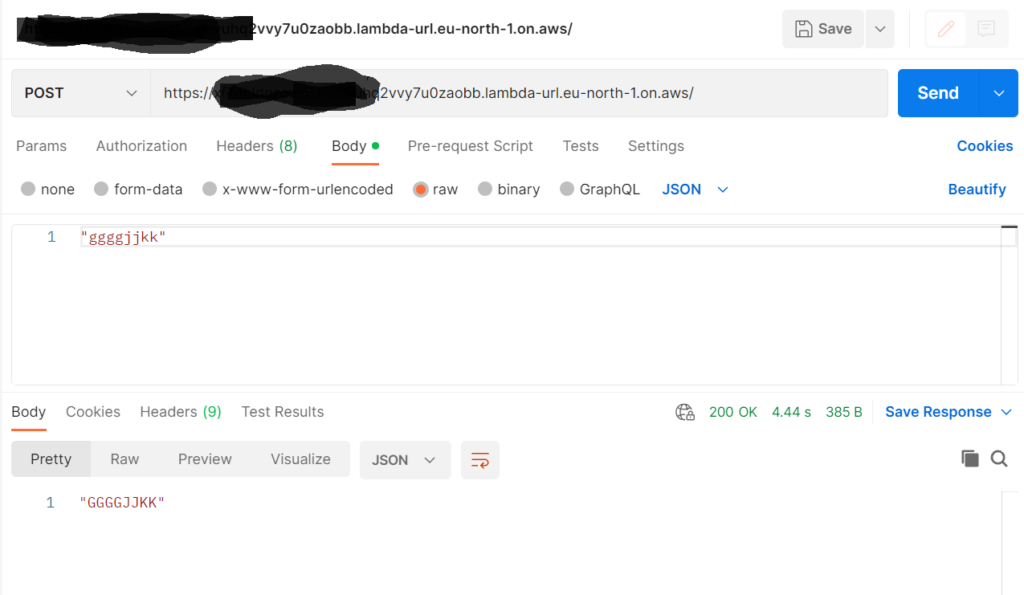
For complete source code: https://github.com/MMahendravarman/Springboot_Examples
Leave a Reply