Spring Boot 3 REST API Doc + Swagger
Springdoc-openapi library helps to automote the generation of REST API documentation .
For Spring boot V3 we need to use springdoc-openapi V2 .
For integration between spring-boot and swagger-ui add the below dependency . Then the swagger will be available at http://server:port/context-path/swagger-ui.html
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.1.0</version>
</dependency>
Sample API:
EmployeeController
@RestController
@RequestMapping("/api/v1/")
@Tag(name = "Employee", description = "the Employee API")
public class EmployeeController {
@Autowired
private EmployeeService svc;
@GetMapping("/employees")
public List<Employee> getAllEmployees() {
return svc.getall();
}
@Operation(summary = "To add an employee", tags = { "Employee" })
@ApiResponses(value = { @ApiResponse(description = "successful operation", content = { @Content(mediaType = "application/json", schema = @Schema(implementation = Employee.class)), @Content(mediaType = "application/xml", schema = @Schema(implementation = Employee.class)) }) })
@PostMapping(value="employee/add",consumes = { "application/json" })
public Employee addEmp(@RequestBody Employee emp) {
return svc.add(emp);
}
}
Employee Model
@Entity
@Data
@NoArgsConstructor
@AllArgsConstructor
@JacksonXmlRootElement(localName = "employee")
@XmlRootElement(name = "employee")
@XmlAccessorType(XmlAccessType.FIELD)
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@JsonProperty("id")
@JacksonXmlProperty(localName = "id")
private Long employeeId;
@JsonProperty("name")
@JacksonXmlProperty(localName = "name")
String name;
@JsonProperty("department")
@JacksonXmlProperty(localName = "department")
String department;
}
After running the application we can view the swagger using below url
http://localhost:8080/swagger-ui.html
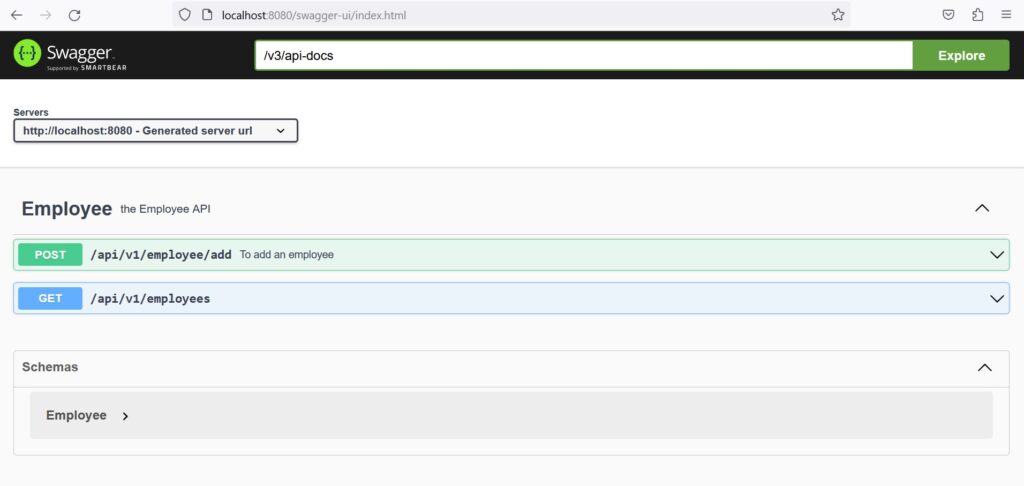
References:
Github URL :
https://github.com/MMahendravarman/Springboot_Examples
Leave a Reply